NextJS Static Site Generation with static exports
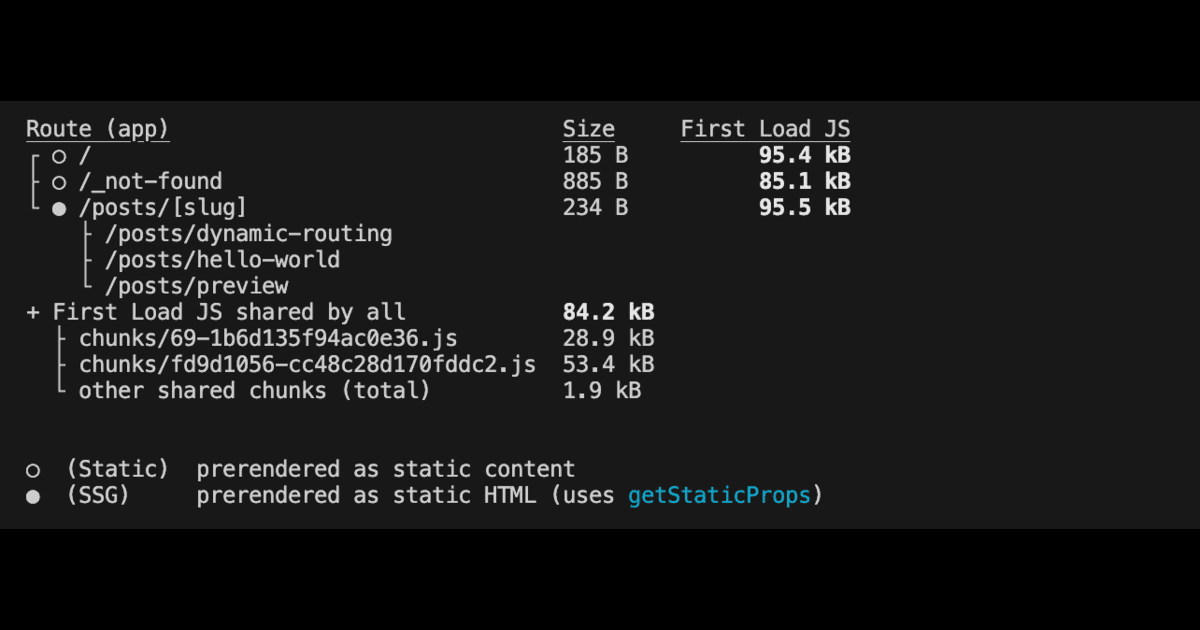
I was looking for a framework to build static website for my blog, and I came across NextJS framework which allows static site generation. In this blog post, we will talk a little bit about what is a static website and which use cases fit with static website. We will also discuss what is NextJS and how does it build static website.
Static Website
Static Website, as the name static implies, is a website which content does not or infrequently changed. Once the website is built, it is done and does not need further processing by the server.
When a user request to view a page from static website, the server, usually via CDN, will serve the page as is to the user.
This approach provides several benefits. The first one is speed. Because your website is already built, your browser does not have to do much processing and simply render it for you.
The second benefit is with Cloud Delivery Network (CDN) caching. When your website is fronted with CDN which is distributed globally, you will take advantage of the caching from the CDN to provide faster response to your user's request.
Last, with your static website, all of the contents are already built. This will allow SEO to easily index your website.
With these benefits, should you build static website? It depends on your use case 🤔. Static Website is good for Blogs, ecommerce, and marketing website, to name a few, because they don't change often. But if your website displays data that change frequently, you will be better off to build a dynamic website with client side rendering or server side rendering.
In my case, I want to build my personal website for blogging, as you can see here. The content will not change that often, therefore I opt-in to build a static website and to build it, I use NextJS.
Static Site Generation with NextJS
NextJS is a web framework utilising React under the hood. It is an open source framework created by Vercel.
NextJS supports different type of rendering, one of them is Static Site Generation (SSG).
With SSG, when you build your website, it will look at your code and generate static pages for your website. It works on both static route and dynamic route.
Let's see it in action
To demonstrate static site generation, let's use the provided blog-starter template and we will walkthrough it.
First, let's create a new NextJS app based on the blog-starter template:
npx create-next-app --example blog-starter blog-starter-app
Open the app folder in your text editor. I use VSCode.
cd blog-starter-app
code -r .
Let's go through the project structure:
_posts
: this folder will contain all of the blog posts.public
: this folder will contain your public assets such as images, icons, etc.src/app
: this folder will contain your website files.
Now, we can run the build command:
npm run build
This command will run next build
to build your HTML pages. Take a look at the output of the build, you can see that some pages are prerendered as static content and some as static HTML.
The differences are, static content pages are pages which do not use any parameters. Whereas static HTML, which generated by SSG, are pages which are dynamic. These pages need to be generated dynamically.
In this example, the /posts/[slug]
pages are dynamically generated based on the number of blog posts that you have. Each posts will be generated as separate HTML page. Take a look at page.tsx
in posts/[slug]
directory, you will find below code snippet
export async function generateStaticParams() {
const posts = getAllPosts();
return posts.map((post) => ({
slug: post.slug,
}));
}
This method is called during build time to get all of available posts and will generate a separate html pages for each posts.
Static Exports
Now, you know how to generate static website with NextJS. So, how can you deploy this static website? First, you need to get your hands on the generated static HTML files. To do that, you can configure NextJS to export the files after they are built.
Add new file called next.config.mjs
in your root directory, and paste in this snippet:
/** @type {import('next').NextConfig} */
const nextConfig = {
output: 'export',
};
export default nextConfig;
When you run npm run build
, this time it will output the generated files into the /out
directory.
You can copy these files and hosted in your prefered web hosting or CDN.
Conclusion
That's it! I hope it explains about static website and how to use NextJS static site generation to generate a static website.
See you next time 👋